使用aapt工具获取apk的信息,可以使用
1 | aapt dump badging xxx.apk |
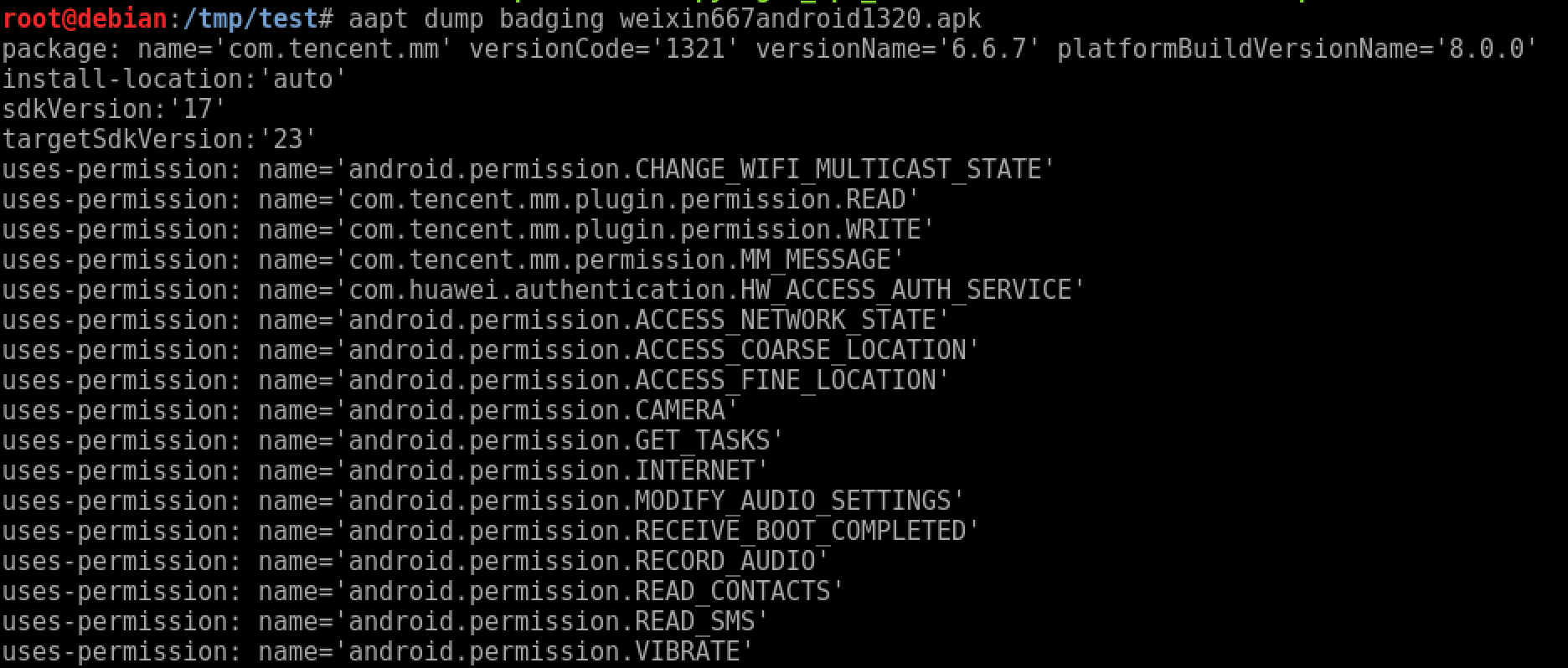
如果没有aapt,使用apt安装:
1 | apt-get install aapt |
写个脚本批量获取
1 | #!/bin/bash |
脚本下载链接:
get_apk_name使用aapt工具获取apk的信息,可以使用
1 | aapt dump badging xxx.apk |
如果没有aapt,使用apt安装:
1 | apt-get install aapt |
写个脚本批量获取
1 | #!/bin/bash |
脚本下载链接:
get_apk_name随着技术的发展,近年很多的网站、移动应用程序、微信小程序等等,采用前后端分离、使用JSON API数据传递这种方式来设计和开发。如此,前端程序可以保持独立、并且相对来说比较完整,后端程序逻辑复杂、性能强劲。前端可以有丰富的对数据的展现方式(图表、动画等等),后端也只需要完善相应的对模型的控制,同时输出一份前端所需要的数据,采用Ajax+JSON方式传递,从而让前后端的开发相对而言都更为轻松,在结构合理、技术完备的情况下,工程层面也能实现较大程度的规模上的突破。
Rails作为一种流行的MVC框架,本身在API的开发上已经有了很成熟的配套设施了。Rails的模板支持.json.jbuilder形式的JSON数据渲染,配合jbuilder从模型对象数组直接创建JSON数据,在开发上有着无与伦比的便利。在此之前,如果使用php开发程序,从数组创建JSON,需要先整理好数组,再json_encode(Array)进行输出,很是麻烦。当然身为PHP程序员的你可能要说,PHP也有很强大的API构建框架,可以很方便的构建出健壮的API,是的,我承认有这些框架的存在,只是限于本人的水平和喜好,在PHP开发方面并没有走的那么深远,也就不熟悉其中的一些高级操作了。如果有兴趣,也可以发邮件就这方面进行一些探讨。
近期由于一个项目的需要,需要从已有的Rails应用中开辟出一个新的独立接口,供客户端程序调用,因此学习了一下Rails API的构建方法,也顺便留下此文,作为记录。关于Rails API的构建,我参考了这篇文章 https://blog.csdn.net/li_001/article/details/65937664
以下就是如何使用Rails构建JSON API
我当前采用的环境是 Ruby 2.4.1 + Rails 5.2.0
首先假设我们的应用已经创建好了,并且建立一个Article模型,包含两个字段,title和content,运行迁移并创建种子数据。
此时进入项目根目录,新建一个控制器:
1 | rails g controller api/v1/base --no-assets |
这个控制器的代码就会位于app/controllers/api/v1/base_controller.rb,我们需要在其中完善一些权限有关的配置。
由于Rails默认的安全属性,以及我们如果之前自定义了一些控制器中的过滤方法,我们需要在该控制器中跳过它,例如我们首先禁用rails自带的CSRF 保护和CSRF token的输出,以及去除掉返回包中的set-cookies头,由于我的应用还使用了devise作为用户认证的模块,所以还要在此禁用掉authenticate_user方法。
完整的代码如下:
1 | class Api::V1::BaseController < ApplicationController |
创建好base控制器以后,我们需要在这个控制器的基础上派生出一些新的控制器,这里我们新建一个articles控制器
1 | rails g controller api/v1/articles --no-assets |
此时除了会生成控制器代码以外,还会在app/views/api/v1目录下创建articles目录,作为模板存放的目录。
articles控制器的代码位于app/controllers/api/v1/users_controller.rb,内容如下
先在其中定义一个show方法:
1 | class Api::V1::ArticlesController < Api::V1::BaseController |
接下来我们对路由config/routes.rb进行配置
1 | namespace :api do |
articles控制器的视图代码位于app/views/api/v1/articles/,我们在其中建立show.json.jbuilder文件,内容如下:
1 | json.article do |
上面这段代码,是jbuilder建立json的语句代码,至于jbuilder的用法,可以参考这篇文章http://ohcoder.com/blog/2015/04/19/jbuilder/
启动Rails程序,访问/api/v1/articles/1.json,即可看到接口输出的JSON数据。
除了从接口获取JSON数据以外,我们还需要向接口POST JSON数据,并希望接口实现数据的解析和保存。
首先像往常一样,需要在控制器中创建create方法,用于新建数据:
1 | def create |
呵呵,一行代码,是的,就这么简单。
而article_params,则是个私有方法,大概需要这样实现:
1 | def article_params |
在客户端(或网页中)向该接口发送数据,地址是/api/v1/articles
发送的数据类似下面这样:
1 | { |
直接发送这条数据可能会出错,因为在请求的时候需要带上json格式描述
1 | Content-Type: application/json |
完整的POST请求,参考下:
1 | POST /api/v1/articles HTTP/1.1 |
Devise是Rails中一个功能强大、逻辑复杂的用于实现站点用户管理和登录的组件。鉴于Ruby之不重复造轮子的精神思想,Devise是值得去深入研究学习一下的。由于Devise本身的复杂性,这里对搭建的过程做一个记录,也借此分享一下基于Devise实现最基本的站点用户管理和登录的过程。
需要在机器上安装Ruby和Rails。
本次编程环境:
首先新建一个Rails项目TestSite,创建Post scaffold,包含title和body两个字段
1 | rails new TestSite |
接着在Gemfile中添加devise
1 | gem 'devise' |
在项目中执行bundle,以及执行devise:install
1 | bundle |
由于我们暂时只在本地测试,因此修改config/environments/development.rb文件,在其中加入mailer配置:
1 | config.action_mailer.default_url_options = { host: 'localhost', port: 3000 } |
指定Devise将要运行在哪个模型上,这里使用user作为模型,那么运行:
1 | rails generate devise User |
下一步,就是运行迁移:
1 | rails db:migrate |
如果希望在应用运行之前校验用户身份,在ApplicationController中加入以下过滤器:
1 | before_action :authenticate_user! |
指定路由:
1 | root to: 'posts#index' |
在Application的首页,加上用户注销的链接:
1 | <%= link_to "Logout", destroy_user_session_path, method: "DELETE" if current_user %> |
如果想要自定义登陆界面的视图,则运行以下命令:
1 | rails g devise:views users |
这里后面带模型名称,就会创建在这个模型范围内的视图(建议这样做,便于以后拓展多重用户身份模型)
此时就可以在相应的视图中自定义登录、注册、重置密码等相应的界面了。
生成控制器就和生成视图的流程是类似的,运行:
1 | rails generate devise:controllers users |
这样控制器就创建好了。
控制器这一段是值得好好说道说道的。
首先观察下控制器,控制器是可以追加功能的,也可以覆盖原来的方法,不过不推荐这样做,因为从目前经验来看devise自带的控制器已经足够健壮了。但是有时候,我们需要在原有的基础上新增一些功能,例如记录登录日志、增加一点自定义认证方式等等。这里简单介绍下如何在控制器中新增功能。
上一步操作,为我们生成了以下控制器:
1 | app/controllers/users/confirmations_controller.rb |
这里以登录为例,如果我们想要改写登录功能,首先我们要在路由中改写我们要复写的控制器路由:
routes.rb中:
1 | Rails.application.routes.draw do |
接着修改app/controllers/users/sessions_controller.rb,这里我们简单实现一个功能,当用户在前台登陆时,在后台的console中输出用户的登录信息。复写create方法:
1 | # POST /resource/sign_in |
尝试在前台登录,控制台输出了我们想要的信息:
待续
上手Markdown,你只需要先熟悉以下几种语法就够了:
下面开始一一讲解
标题很简单,用#号就可以。
一级标题是#,二级标题就用##,三级标题就用###,以此类推。
例如以下内容:
# 一级标题
## 二级标题
### 三级标题
在页面中展示的样子就是:
至于章节,一般用—来表示,这样在页面中会显示章节线。
例如:
—
Area 1
---
Area 2
效果就是:
Area 1
Area 2
粗体用**内容**
来表示,斜体用*内容*
来表示。
例如:
*斜体*
**粗体**
效果:
斜体
粗体
列表一般用
+ 无序列表1级
+ 无序列表2级
+ 无序列表3级
1. 有序列表1
2. 有序列表2
来表示
显示的效果如下:
1 |
|
显示的效果就是:
1 | #test code |
这个稍微复杂点,链接用:
[链接描述](URL)
图片用

例如:
[百度](https://www.baidu.com/)

效果:
表格一般是以下形式:
|表格|表格|表格|表格|表格|
|:-|:-|:-|:-|:-|
|1|2|3|4|5
|6|7|8|9|0
效果:
表格 | 表格 | 表格 | 表格 | 表格 |
---|---|---|---|---|
1 | 2 | 3 | 4 | 5 |
6 | 7 | 8 | 9 | 0 |
1 | ~# hexo new "Post Title" |
Use keyword tag codeblock and endcodeblock
1 | {% codeblock lang:javascript %} |
Or just use three backquotes
1 | \`\`\`[language] [title] [url] [link text] |
Images
1 | {% img [class names] /path/to/image [width] [height] [title text [alt text]] %} |
Links
1 | {% link text url [external] [title] %} |
In _config.yml, set post_asset_folder as true
1 | post_asset_folder: true |
Then your _posts directory maybe such as this
1 | root@debian:~/chorder.net/source# tree _posts/ |
You can directly include images from resources directory:
1 | {% asset_img xxx.jpg ALT TITLE %} |
Your may want to organize your posts as different categories and tags.
First you need to define categories and tags directories in _config.yml, default is like this
1 | # Directory |
Then you can generate a new page to navigate the posts
1 | ~# hexo new page "categories" |
After that, the tags and categories directory will being created in source:
1 | root@debian:~/chorder.net/source# tree |
And on the next time when you creating a new post, you could statement the category and tags of your post,
on the head of post markdown file, just like:
1 | --- |
The archives of tags and categories will showing in the site automatically.
In your hexo directory, run
1 | ~# npm install hexo-server --save |
Then start the server:
1 | ~# hexo server -p PORT |
Or if your want publish static pages as an production envirement, you should first generate static files:
1 | ~# hexo generate |
And then start the server as static mode, it will only deploy pages which included in public directory:
1 | ~# hexo server -s |
1 | {% include_code [title] [lang:language] path/to/file %} |
1 | {% youtube video_id %} |
1 | {% vimeo video_id %} |
1 | # article |
As for optimize your site with a better SEO, ensure you has create sitemap:
1 | #for google sitemap, install this package |
Over that, tell the spider what you have done, write this in your robots.txt, and save it at source directory:
1 | Sitemap: http://YOUR DOMAIN/sitemap.xml |
Example robots.txt as mine:
1 | User-agent: * |
You can see both above at:
Sitemap Baidu Sitemap robots.txt1 | loop do |
Talk is cheap, show me the code.
A new hexo blog, themed by alpha-dust(Jonathan Klughertz)
by Chorder, May 10, 2018.
put it to your MSFPATH/modules/exploits/multi/http/
struts2_s2045_rce.rb:
1 | require 'msf/core' |
错误详情:
1 | ruby-2.3.3 - #compiling.......................................................................- |
查看/usr/local/rvm/log/1488041042_ruby-2.3.3/make.log发现是openssl版本过老导致的。
解决方案:
第一步:先安装用于rvm的openssl:
1 | rvm pkg install openssl |
第二步:编译安装ruby,指定openssl目录(我的是/usr/local/rvm/usr/)
1 | rvm install ruby-2.3.3 --with-openssl-dir=/usr/local/rvm/usr/ |
PostgreSQL其实并不是一定需要通过端口来连接。
在PostgreSQL的配置中有一项是将PSQL配置成不开放端口,只使用Unix套接字的通信。
具体的内容,可以参考下国内PostgreSQL大神的博客:
https://github.com/digoal/blog/blob/master/201701/20170111_01.md
也就是,在postgresql.conf中,将监听的主机listen_address改成空,即可在不开启端口的情况下进行通信。
如果你的 unix_socket_directories 选项配置的路径是在/tmp,那么在postgresql运行后,你的/tmp下面将会有”.s.PGSQL.5432”这个文件(通过ls -a /tmp 可以查看到)。
如果在配置好listen_address和unix_socket_directories之后,重启postgresql服务,并且/tmp下的套接字文件存在,就说已经配置好。
这时在metasploit的database.yml文件中,配置host为/tmp,用户名和密码可以随便写,数据库名称就写metasploit的数据库。
然后再配置一下postgresql的”pg_hba.conf”文件,
在其中加入local all all trust
将所有来自本地unix域的请求设置为信任。就可以在不开端口的情况下在metasploit中使用postgresql了。
为了安全起见,还可以在
postgresql.conf中,将unix_socket_permissions改为0770,这样可以避免无关用户滥用这个unix接口。
不知原文出自哪里,如果您是作者,请私信我补充来源链接。
记录一些排查常见日志的命令,方法wiki,欢迎补充(Markdown 语法)。
1 | cat 2015_7_25_test_access.log | grep "sqlmap" | wc -l |
1 | sed -i '/Indy Library/d' 2015_7_25_test_access.log |
1 | find ./ -name "*.log" |xargs grep "sqlmap" |wc -l |
1 | cat cszl988.log | awk '{print $1}' | awk -F : '{print $2}' | sort -u | wc -l |
1 | grep -E -o "([0-9]{1,3}[\.]){3}[0-9]{1,3}" |
1 | tail 3.log | awk '{print $7}' | sort | uniq -c |
1 | #!/bin/bash |
1 | cat luban.log | grep sqlmap | awk '{print $7}' | xargs python -c 'import sys, urllib; print urllib.unquote(sys.argv[1])' |
1 | [root@pentest temp]# cat luban.log | grep sqlmap | wc -l |
1 | cat luban.log | grep sqlmap | awk '{print $7}' | more |
1 | cat luban.log | grep sqlmap | awk '{print $7}' | xargs python -c 'import sys, urllib; print urllib.unquote(sys.argv[1])' |
Update your browser to view this website correctly. Update my browser now